2024-05-07 Daily Challenge
Today I have done leetcode's May LeetCoding Challenge with cpp
.
May LeetCoding Challenge 7
Description
Double a Number Represented as a Linked List
You are given the head
of a non-empty linked list representing a non-negative integer without leading zeroes.
Return the head
of the linked list after doubling it.
Example 1:
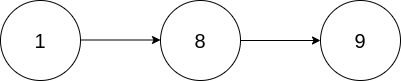
Input: head = [1,8,9] Output: [3,7,8] Explanation: The figure above corresponds to the given linked list which represents the number 189. Hence, the returned linked list represents the number 189 * 2 = 378.
Example 2:
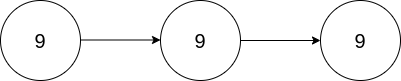
Input: head = [9,9,9] Output: [1,9,9,8] Explanation: The figure above corresponds to the given linked list which represents the number 999. Hence, the returned linked list reprersents the number 999 * 2 = 1998.
Constraints:
- The number of nodes in the list is in the range
[1, 104]
0 <= Node.val <= 9
- The input is generated such that the list represents a number that does not have leading zeros, except the number
0
itself.
Solution
class Solution {
int helper(ListNode *head) {
if(!head) return 0;
head->val *= 2;
if(helper(head->next)) {
head->val += 1;
}
if(head->val > 9) {
head->val -= 10;
return 1;
}
return 0;
}
public:
ListNode* doubleIt(ListNode* head) {
if(helper(head)) {
return new ListNode(1, head);
}
return head;
}
};