2024-09-09 Daily Challenge
Today I have done leetcode's September LeetCoding Challenge with cpp
.
September LeetCoding Challenge 9
Description
Spiral Matrix IV
You are given two integers m
and n
, which represent the dimensions of a matrix.
You are also given the head
of a linked list of integers.
Generate an m x n
matrix that contains the integers in the linked list presented in spiral order (clockwise), starting from the top-left of the matrix. If there are remaining empty spaces, fill them with -1
.
Return the generated matrix.
Example 1:
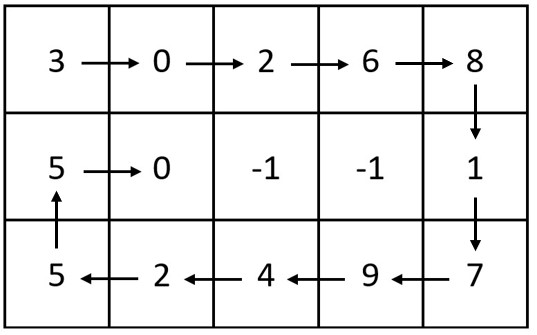
Input: m = 3, n = 5, head = [3,0,2,6,8,1,7,9,4,2,5,5,0] Output: [[3,0,2,6,8],[5,0,-1,-1,1],[5,2,4,9,7]] Explanation: The diagram above shows how the values are printed in the matrix. Note that the remaining spaces in the matrix are filled with -1.
Example 2:
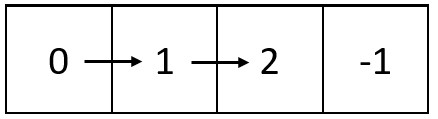
Input: m = 1, n = 4, head = [0,1,2] Output: [[0,1,2,-1]] Explanation: The diagram above shows how the values are printed from left to right in the matrix. The last space in the matrix is set to -1.
Constraints:
1 <= m, n <= 105
1 <= m * n <= 105
- The number of nodes in the list is in the range
[1, m * n]
. 0 <= Node.val <= 1000
Solution
happy birthday to myself~
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
const int MOVES[4][2] = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}};
public:
vector<vector<int>> spiralMatrix(int m, int n, ListNode* head) {
vector<vector<int>> answer(m, vector<int>(n, -1));
int x = 0;
int y = -1;
while(head) {
int move = 0;
for(int i = 0; i < n && head; ++i) {
x += MOVES[move][0];
y += MOVES[move][1];
answer[x][y] = head->val;
head = head->next;
}
move += 1;
for(int i = 0; i < m - 1 && head; ++i) {
x += MOVES[move][0];
y += MOVES[move][1];
answer[x][y] = head->val;
head = head->next;
}
move += 1;
for(int i = 0; i < n - 1 && head; ++i) {
x += MOVES[move][0];
y += MOVES[move][1];
answer[x][y] = head->val;
head = head->next;
}
move += 1;
for(int i = 0; i < m - 2 && head; ++i) {
x += MOVES[move][0];
y += MOVES[move][1];
answer[x][y] = head->val;
head = head->next;
}
n -= 2;
m -= 2;
}
return answer;
}
};