2024-08-11 Daily Challenge
Today I have done leetcode's August LeetCoding Challenge with cpp
.
August LeetCoding Challenge 11
Description
Minimum Number of Days to Disconnect Island
You are given an m x n
binary grid grid
where 1
represents land and 0
represents water. An island is a maximal 4-directionally (horizontal or vertical) connected group of 1
's.
The grid is said to be connected if we have exactly one island, otherwise is said disconnected.
In one day, we are allowed to change any single land cell (1)
into a water cell (0)
.
Return the minimum number of days to disconnect the grid.
Example 1:
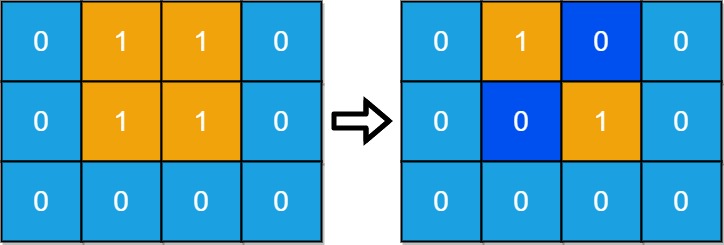
Input: grid = [[0,1,1,0],[0,1,1,0],[0,0,0,0]]Output: 2 Explanation: We need at least 2 days to get a disconnected grid. Change land grid[1][1] and grid[0][2] to water and get 2 disconnected island.
Example 2:
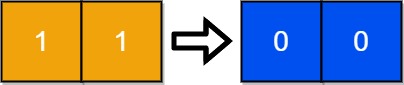
Input: grid = [[1,1]] Output: 2 Explanation: Grid of full water is also disconnected ([[1,1]] -> [[0,0]]), 0 islands.
Constraints:
m == grid.length
n == grid[i].length
1 <= m, n <= 30
grid[i][j]
is either0
or1
.
Solution
class Solution {
const vector<pair<int, int>> MOVES = {
{0, 1},
{1, 0},
{0, -1},
{-1, 0}
};
void dfs(vector<vector<bool>> &seen, const vector<vector<int>> &grid, int rows, int cols, int row, int col) {
seen[row][col] = true;
for(auto [dRow, dCol] : MOVES) {
int newRow = row + dRow;
int newCol = col + dCol;
if(newRow < 0 || newCol < 0 || newRow >= rows || newCol >= cols) continue;
if(!grid[newRow][newCol]) continue;
if(seen[newRow][newCol]) continue;
dfs(seen, grid, rows, cols, newRow, newCol);
}
}
int countIsland(const vector<vector<int>> &grid) {
int rows = grid.size();
int cols = grid.front().size();
vector<vector<bool>> seen(rows, vector<bool>(cols));
int islands = 0;
for(int i = 0; i < rows; ++i) {
for(int j = 0; j < cols; ++j) {
if(seen[i][j] || !grid[i][j]) continue;
islands += 1;
dfs(seen, grid, rows, cols, i, j);
}
}
return islands;
}
public:
int minDays(vector<vector<int>>& grid) {
if(countIsland(grid) != 1) return 0;
for(auto &row : grid) {
for(auto &cell : row) {
if(!cell) continue;
cell = 0;
if(countIsland(grid) != 1) return 1;
cell = 1;
}
}
return 2;
}
};