2024-07-26 Daily Challenge
Today I have done leetcode's July LeetCoding Challenge with cpp
.
July LeetCoding Challenge 26
Description
Find the City With the Smallest Number of Neighbors at a Threshold Distance
There are n
cities numbered from 0
to n-1
. Given the array edges
where edges[i] = [fromi, toi, weighti]
represents a bidirectional and weighted edge between cities fromi
and toi
, and given the integer distanceThreshold
.
Return the city with the smallest number of cities that are reachable through some path and whose distance is at most distanceThreshold
, If there are multiple such cities, return the city with the greatest number.
Notice that the distance of a path connecting cities i and j is equal to the sum of the edges' weights along that path.
Example 1:
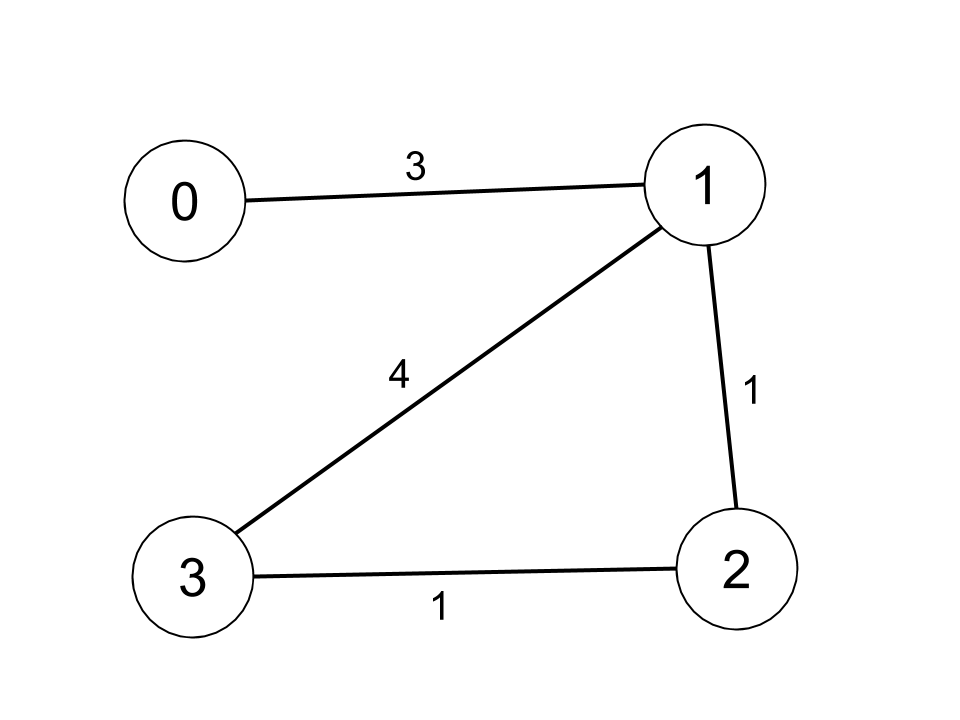
Input: n = 4, edges = [[0,1,3],[1,2,1],[1,3,4],[2,3,1]], distanceThreshold = 4 Output: 3 Explanation: The figure above describes the graph. The neighboring cities at a distanceThreshold = 4 for each city are: City 0 -> [City 1, City 2] City 1 -> [City 0, City 2, City 3] City 2 -> [City 0, City 1, City 3] City 3 -> [City 1, City 2] Cities 0 and 3 have 2 neighboring cities at a distanceThreshold = 4, but we have to return city 3 since it has the greatest number.
Example 2:
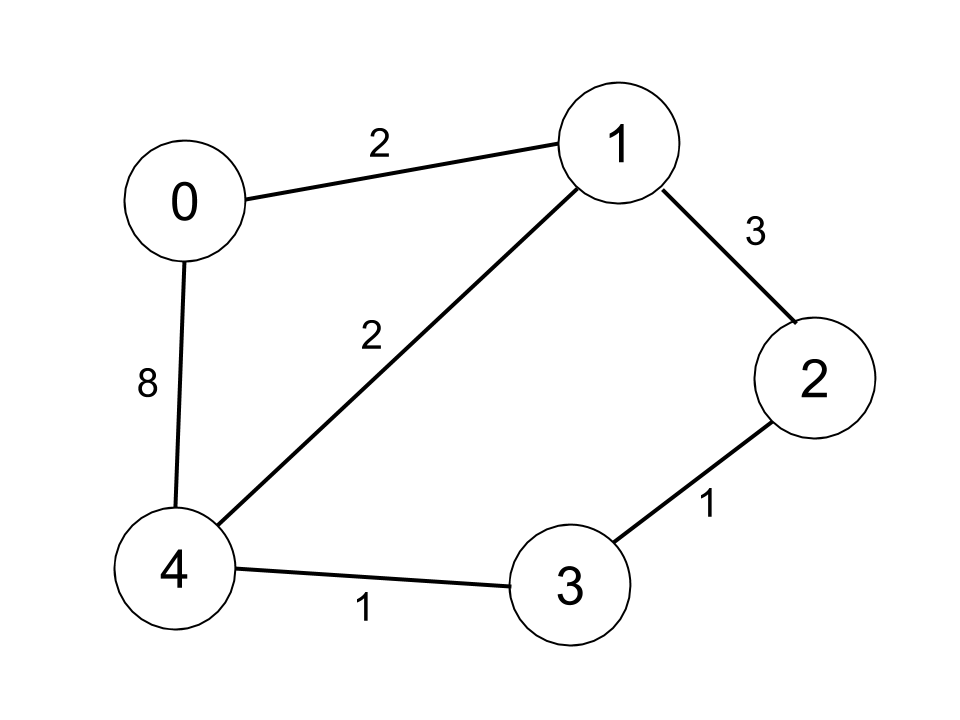
Input: n = 5, edges = [[0,1,2],[0,4,8],[1,2,3],[1,4,2],[2,3,1],[3,4,1]], distanceThreshold = 2 Output: 0 Explanation: The figure above describes the graph. The neighboring cities at a distanceThreshold = 2 for each city are: City 0 -> [City 1] City 1 -> [City 0, City 4] City 2 -> [City 3, City 4] City 3 -> [City 2, City 4] City 4 -> [City 1, City 2, City 3] The city 0 has 1 neighboring city at a distanceThreshold = 2.
Constraints:
2 <= n <= 100
1 <= edges.length <= n * (n - 1) / 2
edges[i].length == 3
0 <= fromi < toi < n
1 <= weighti, distanceThreshold <= 10^4
- All pairs
(fromi, toi)
are distinct.
Solution
class Solution {
using pi = pair<int, int>;
int reachedNeighbors(const vector<vector<pi>> &neighbors, int start, int n, int distanceThreshold) {
vector<bool> visit(n);
priority_queue<pi, vector<pi>, greater<pi>> pq;
pq.push({0, start});
int result = -1; // remove itself in advance
while(pq.size()) {
auto [d, current] = pq.top();
pq.pop();
if(visit[current]) continue;
result += 1;
visit[current] = true;
for(auto &[next, p] : neighbors[current]) {
if(p + d <= distanceThreshold) {
pq.push({p + d, next});
}
}
}
return result;
}
public:
int findTheCity(int n, vector<vector<int>>& edges, int distanceThreshold) {
vector<vector<pi>> neighbors(n);
for(const auto &edge : edges) {
neighbors[edge[0]].push_back({edge[1], edge[2]});
neighbors[edge[1]].push_back({edge[0], edge[2]});
}
int count = INT_MAX;
int answer = n - 1;
for(int i = n - 1; i >= 0; --i) {
int reached = reachedNeighbors(neighbors, i, n, distanceThreshold);
if(reached < count) {
count = reached;
answer = i;
}
}
return answer;
}
};