2024-07-15 Daily Challenge
Today I have done leetcode's July LeetCoding Challenge with cpp
.
July LeetCoding Challenge 15
Description
Create Binary Tree From Descriptions
You are given a 2D integer array descriptions
where descriptions[i] = [parenti, childi, isLefti]
indicates that parenti
is the parent of childi
in a binary tree of unique values. Furthermore,
- If
isLefti == 1
, thenchildi
is the left child ofparenti
. - If
isLefti == 0
, thenchildi
is the right child ofparenti
.
Construct the binary tree described by descriptions
and return its root.
The test cases will be generated such that the binary tree is valid.
Example 1:
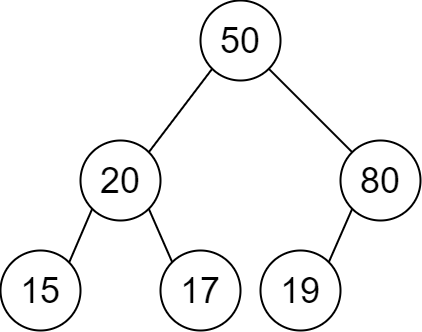
Input: descriptions = [[20,15,1],[20,17,0],[50,20,1],[50,80,0],[80,19,1]] Output: [50,20,80,15,17,19] Explanation: The root node is the node with value 50 since it has no parent. The resulting binary tree is shown in the diagram.
Example 2:
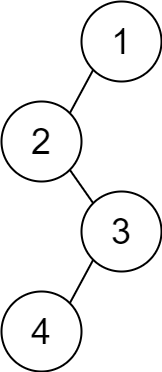
Input: descriptions = [[1,2,1],[2,3,0],[3,4,1]] Output: [1,2,null,null,3,4] Explanation: The root node is the node with value 1 since it has no parent. The resulting binary tree is shown in the diagram.
Constraints:
1 <= descriptions.length <= 104
descriptions[i].length == 3
1 <= parenti, childi <= 105
0 <= isLefti <= 1
- The binary tree described by
descriptions
is valid.
Solution
class Solution {
public:
TreeNode* createBinaryTree(vector<vector<int>>& descriptions) {
map<int, pair<int, int>> child;
map<int, bool> notRoot;
for(const auto &description : descriptions) {
if(description[2]) {
child[description[0]].first = description[1];
} else {
child[description[0]].second = description[1];
}
notRoot[description[1]] = true;
}
queue<TreeNode *> q;
int rootN;
for(const auto &description : descriptions) {
if(!notRoot[description[0]]) rootN = description[0];
}
TreeNode *root = new TreeNode(rootN);
q.push(root);
while(q.size()) {
auto current = q.front();
q.pop();
if(child[current->val].first) {
current->left = new TreeNode(child[current->val].first);
q.push(current->left);
}
if(child[current->val].second) {
current->right = new TreeNode(child[current->val].second);
q.push(current->right);
}
}
return root;
}
};