2024-05-13 Daily Challenge
Today I have done leetcode's May LeetCoding Challenge with cpp
.
May LeetCoding Challenge 13
Description
Score After Flipping Matrix
You are given an m x n
binary matrix grid
.
A move consists of choosing any row or column and toggling each value in that row or column (i.e., changing all 0
's to 1
's, and all 1
's to 0
's).
Every row of the matrix is interpreted as a binary number, and the score of the matrix is the sum of these numbers.
Return the highest possible score after making any number of moves (including zero moves).
Example 1:
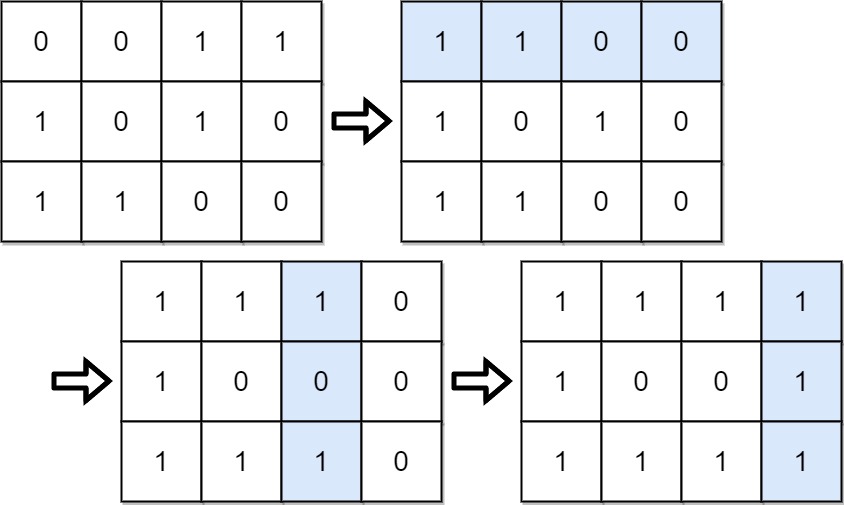
Input: grid = [[0,0,1,1],[1,0,1,0],[1,1,0,0]] Output: 39 Explanation: 0b1111 + 0b1001 + 0b1111 = 15 + 9 + 15 = 39
Example 2:
Input: grid = [[0]] Output: 1
Constraints:
m == grid.length
n == grid[i].length
1 <= m, n <= 20
grid[i][j]
is either0
or1
.
Solution
class Solution {
public:
int matrixScore(vector<vector<int>>& grid) {
int n = grid.size();
int m = grid.front().size();
int answer = (1 << (m - 1)) * n;
for(int j = 1; j < m; ++j) {
int value = 1 << (m - 1 - j);
int same = 0;
for(int i = 0; i < n; ++i) {
same += (grid[i][j] == grid[i][0]);
}
answer += max(same, n - same) * value;
}
return answer;
}
};