2024-02-28 Daily Challenge
Today I have done leetcode's February LeetCoding Challenge with cpp
.
February LeetCoding Challenge 28
Description
Find Bottom Left Tree Value
Given the root
of a binary tree, return the leftmost value in the last row of the tree.
Example 1:
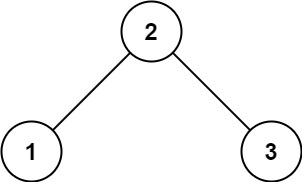
Input: root = [2,1,3] Output: 1
Example 2:
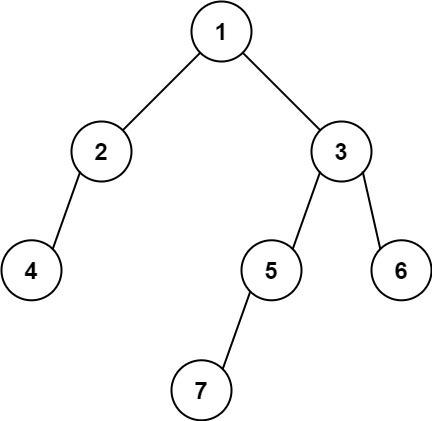
Input: root = [1,2,3,4,null,5,6,null,null,7] Output: 7
Constraints:
- The number of nodes in the tree is in the range
[1, 104]
. -231 <= Node.val <= 231 - 1
Solution
auto speedup = [](){
cin.tie(nullptr);
cout.tie(nullptr);
ios::sync_with_stdio(false);
return 0;
}();
class Solution {
pair<int, int> solve(TreeNode *root, int level) {
if(!root) return {-1, -1};
auto [llevel, lvalue] = solve(root->left, level + 1);
auto [rlevel, rvalue] = solve(root->right, level + 1);
if(llevel == -1 && rlevel == -1) return {level, root->val};
if(llevel >= rlevel) return {llevel, lvalue};
return {rlevel, rvalue};
}
public:
int findBottomLeftValue(TreeNode* root) {
return solve(root, 0).second;
}
};
// Accepted
// 77/77 cases passed (11 ms)
// Your runtime beats 42.78 % of cpp submissions
// Your memory usage beats 96.27 % of cpp submissions (20 MB)