2024-02-26 Daily Challenge
Today I have done leetcode's February LeetCoding Challenge with cpp
.
February LeetCoding Challenge 26
Description
Same Tree
Given the roots of two binary trees p
and q
, write a function to check if they are the same or not.
Two binary trees are considered the same if they are structurally identical, and the nodes have the same value.
Example 1:
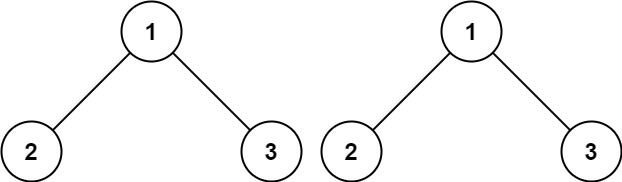
Input: p = [1,2,3], q = [1,2,3] Output: true
Example 2:
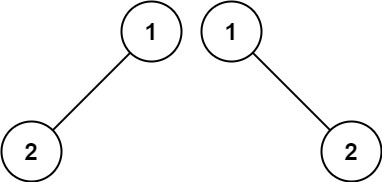
Input: p = [1,2], q = [1,null,2] Output: false
Example 3:
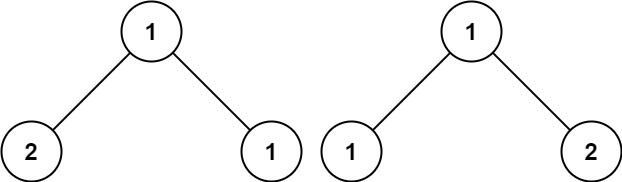
Input: p = [1,2,1], q = [1,1,2] Output: false
Constraints:
- The number of nodes in both trees is in the range
[0, 100]
. -104 <= Node.val <= 104
Solution
class Solution {
public:
bool isSameTree(TreeNode* p, TreeNode* q) {
if(p == q) return true;
if(!p || !q) return false;
if(p->val != q->val) return false;
return isSameTree(p->left, q->left) && isSameTree(p->right, q->right);
}
};
// Accepted
// 63/63 cases passed (0 ms)
// Your runtime beats 100 % of cpp submissions
// Your memory usage beats 25.4 % of cpp submissions (11.6 MB)