2023-12-13 Daily Challenge
Today I have done leetcode's December LeetCoding Challenge with cpp
.
December LeetCoding Challenge 13
Description
Special Positions in a Binary Matrix
Given an m x n
binary matrix mat
, return the number of special positions in mat
.
A position (i, j)
is called special if mat[i][j] == 1
and all other elements in row i
and column j
are 0
(rows and columns are 0-indexed).
Example 1:
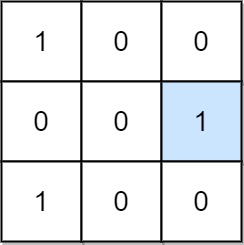
Input: mat = [[1,0,0],[0,0,1],[1,0,0]] Output: 1 Explanation: (1, 2) is a special position because mat[1][2] == 1 and all other elements in row 1 and column 2 are 0.
Example 2:
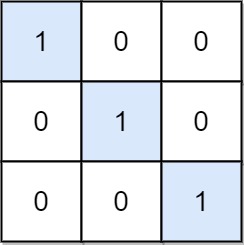
Input: mat = [[1,0,0],[0,1,0],[0,0,1]] Output: 3 Explanation: (0, 0), (1, 1) and (2, 2) are special positions.
Constraints:
m == mat.length
n == mat[i].length
1 <= m, n <= 100
mat[i][j]
is either0
or1
.
Solution
class Solution {
public:
int numSpecial(vector<vector<int>>& mat) {
int rows = mat.size();
int cols = mat.front().size();
vector<int> rowCount(rows);
vector<int> colCount(cols);
for(int i = 0; i < rows; ++i) {
for(int j = 0; j < cols; ++j) {
if(mat[i][j]) {
rowCount[i] += 1;
colCount[j] += 1;
}
}
}
int answer = 0;
for(int i = 0; i < rows; ++i) {
if(rowCount[i] != 1) continue;
for(int j = 0; j < cols; ++j) {
if(!mat[i][j] || colCount[j] != 1) continue;
answer += 1;
}
}
return answer;
}
};
// Accepted
// 95/95 cases passed (13 ms)
// Your runtime beats 83.42 % of cpp submissions
// Your memory usage beats 12.89 % of cpp submissions (13.4 MB)