2023-10-24 Daily Challenge
Today I have done leetcode's October LeetCoding Challenge with cpp
.
October LeetCoding Challenge 24
Description
Find Largest Value in Each Tree Row
Given the root
of a binary tree, return an array of the largest value in each row of the tree (0-indexed).
Example 1:
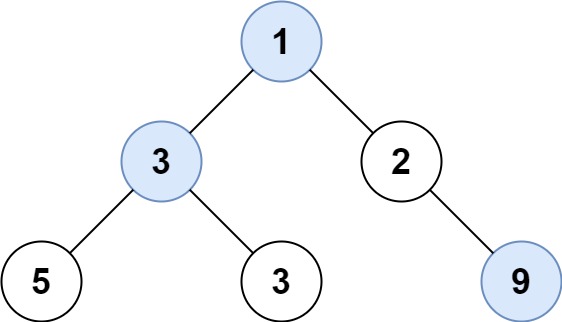
Input: root = [1,3,2,5,3,null,9] Output: [1,3,9]
Example 2:
Input: root = [1,2,3] Output: [1,3]
Constraints:
- The number of nodes in the tree will be in the range
[0, 104]
. -231 <= Node.val <= 231 - 1
Solution
class Solution {
public:
vector<int> largestValues(TreeNode* root) {
if(!root) return {};
vector<int> answer;
queue<TreeNode *> q;
q.push(root);
while(q.size()) {
int sz = q.size();
int maximum = INT_MIN;
for(int _ = 0; _ < sz; ++_) {
auto current = q.front();
q.pop();
maximum = max(maximum, current->val);
if(current->left) {
q.push(current->left);
}
if(current->right) {
q.push(current->right);
}
}
answer.push_back(maximum);
}
return answer;
}
};
// Accepted
// 78/78 cases passed (8 ms)
// Your runtime beats 68.56 % of cpp submissions
// Your memory usage beats 50.91 % of cpp submissions (22.5 MB)