2023-10-17 Daily Challenge
Today I have done leetcode's October LeetCoding Challenge with cpp
.
October LeetCoding Challenge 17
Description
Validate Binary Tree Nodes
You have n
binary tree nodes numbered from 0
to n - 1
where node i
has two children leftChild[i]
and rightChild[i]
, return true
if and only if all the given nodes form exactly one valid binary tree.
If node i
has no left child then leftChild[i]
will equal -1
, similarly for the right child.
Note that the nodes have no values and that we only use the node numbers in this problem.
Example 1:
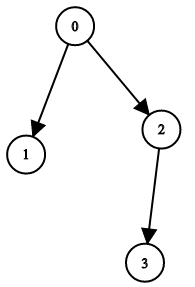
Input: n = 4, leftChild = [1,-1,3,-1], rightChild = [2,-1,-1,-1] Output: true
Example 2:
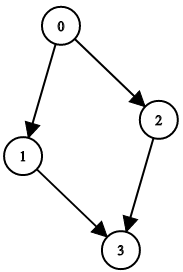
Input: n = 4, leftChild = [1,-1,3,-1], rightChild = [2,3,-1,-1] Output: false
Example 3:
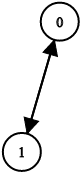
Input: n = 2, leftChild = [1,0], rightChild = [-1,-1] Output: false
Constraints:
n == leftChild.length == rightChild.length
1 <= n <= 104
-1 <= leftChild[i], rightChild[i] <= n - 1
Solution
auto speedup = [](){
cin.tie(nullptr);
cout.tie(nullptr);
ios::sync_with_stdio(false);
return 0;
}();
class Solution {
public:
bool validateBinaryTreeNodes(int n, vector<int>& leftChild, vector<int>& rightChild) {
vector<bool> root(n, true);
for(int i = 0; i < n; ++i) {
if(leftChild[i] != -1) root[leftChild[i]] = false;
if(rightChild[i] != -1) root[rightChild[i]] = false;
}
queue<int> q;
for(int i = 0; i < n; ++i) {
if(root[i]) q.push(i);
}
if(q.size() != 1) return false;
set<int> visit;
while(q.size()) {
auto current = q.front();
q.pop();
if(visit.count(current)) return false;
visit.insert(current);
if(leftChild[current] != -1) {
q.push(leftChild[current]);
}
if(rightChild[current] != -1) {
q.push(rightChild[current]);
}
}
return visit.size() == n;
}
};
// Accepted
// 44/44 cases passed (50 ms)
// Your runtime beats 46.81 % of cpp submissions
// Your memory usage beats 42.86 % of cpp submissions (37.5 MB)