2023-08-15 Daily Challenge
Today I have done leetcode's August LeetCoding Challenge with cpp
.
August LeetCoding Challenge 15
Description
Partition List
Given the head
of a linked list and a value x
, partition it such that all nodes less than x
come before nodes greater than or equal to x
.
You should preserve the original relative order of the nodes in each of the two partitions.
Example 1:
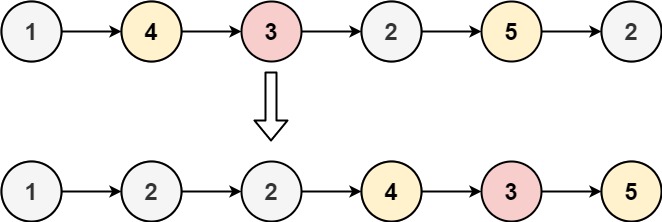
Input: head = [1,4,3,2,5,2], x = 3 Output: [1,2,2,4,3,5]
Example 2:
Input: head = [2,1], x = 2 Output: [1,2]
Constraints:
- The number of nodes in the list is in the range
[0, 200]
. -100 <= Node.val <= 100
-200 <= x <= 200
Solution
class Solution {
public:
ListNode* partition(ListNode* head, int x) {
ListNode *newFront = new ListNode(-1);
ListNode *frontTail = newFront;
ListNode *newBack = new ListNode(-1);
ListNode *backTail = newBack;
while(head) {
if(head->val < x) {
frontTail->next = head;
frontTail = frontTail->next;
} else {
backTail->next = head;
backTail = backTail->next;
}
head = head->next;
}
backTail->next = nullptr;
frontTail->next = newBack->next;
ListNode* answer = newFront->next;
delete newFront;
delete newBack;
return answer;
}
};
// Accepted
// 168/168 cases passed (7 ms)
// Your runtime beats 73.71 % of cpp submissions
// Your memory usage beats 32.53 % of cpp submissions (10.3 MB)