2023-07-10 Daily Challenge
Today I have done leetcode's July LeetCoding Challenge with cpp
.
July LeetCoding Challenge 10
Description
Minimum Depth of Binary Tree
Given a binary tree, find its minimum depth.
The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.
Note: A leaf is a node with no children.
Example 1:
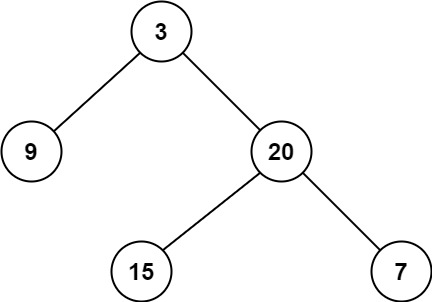
Input: root = [3,9,20,null,null,15,7] Output: 2
Example 2:
Input: root = [2,null,3,null,4,null,5,null,6] Output: 5
Constraints:
- The number of nodes in the tree is in the range
[0, 105]
. -1000 <= Node.val <= 1000
Solution
auto speedup = [](){
cin.tie(nullptr);
cout.tie(nullptr);
ios::sync_with_stdio(false);
return 0;
}();
class Solution {
public:
int minDepth(TreeNode* root) {
if (root == NULL) return 0;
queue<TreeNode*> Q;
Q.push(root);
int i = 0;
while (!Q.empty()) {
i++;
int k = Q.size();
for (int j = 0; j < k; ++j) {
TreeNode* rt = Q.front();
Q.pop();
if (rt->left) Q.push(rt->left);
if (rt->right) Q.push(rt->right);
if (rt->left == NULL && rt->right == NULL) return i;
}
}
return -1; //For the compiler thing. The code never runs here.
}
};
// Accepted
// 52/52 cases passed (237 ms)
// Your runtime beats 99.27 % of cpp submissions
// Your memory usage beats 99.31 % of cpp submissions (144.4 MB)