2023-03-14 Daily Challenge
Today I have done leetcode's March LeetCoding Challenge with cpp
.
March LeetCoding Challenge 14
Description
Sum Root to Leaf Numbers
You are given the root
of a binary tree containing digits from 0
to 9
only.
Each root-to-leaf path in the tree represents a number.
- For example, the root-to-leaf path
1 -> 2 -> 3
represents the number123
.
Return the total sum of all root-to-leaf numbers. Test cases are generated so that the answer will fit in a 32-bit integer.
A leaf node is a node with no children.
Example 1:
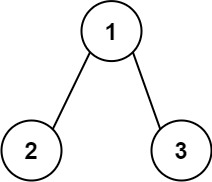
Input: root = [1,2,3] Output: 25 Explanation: The root-to-leaf path1->2
represents the number12
. The root-to-leaf path1->3
represents the number13
. Therefore, sum = 12 + 13 =25
.
Example 2:
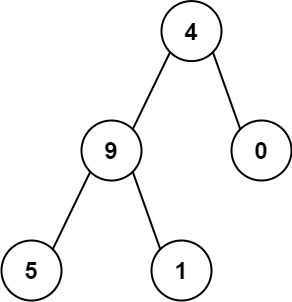
Input: root = [4,9,0,5,1] Output: 1026 Explanation: The root-to-leaf path4->9->5
represents the number 495. The root-to-leaf path4->9->1
represents the number 491. The root-to-leaf path4->0
represents the number 40. Therefore, sum = 495 + 491 + 40 =1026
.
Constraints:
- The number of nodes in the tree is in the range
[1, 1000]
. 0 <= Node.val <= 9
- The depth of the tree will not exceed
10
.
Solution
class Solution {
public:
int sumNumbers(TreeNode* root, int cur = 0) {
if(!root) return 0;
cur = cur * 10 + root->val;
if(root->left || root->right) return sumNumbers(root->left, cur) + sumNumbers(root->right, cur);
return cur;
}
};
// Accepted
// 108/108 cases passed (0 ms)
// Your runtime beats 100 % of cpp submissions
// Your memory usage beats 80.09 % of cpp submissions (9.1 MB)