2022-11-15 Daily Challenge
Today I have done leetcode's November LeetCoding Challenge with cpp
.
November LeetCoding Challenge 15
Description
Count Complete Tree Nodes
Given the root
of a complete binary tree, return the number of the nodes in the tree.
According to Wikipedia, every level, except possibly the last, is completely filled in a complete binary tree, and all nodes in the last level are as far left as possible. It can have between 1
and 2h
nodes inclusive at the last level h
.
Design an algorithm that runs in less than O(n)
time complexity.
Example 1:
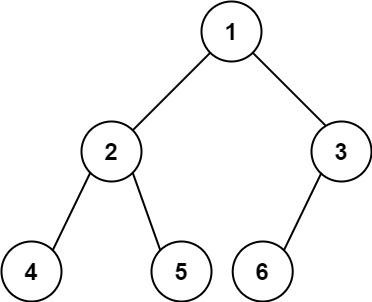
Input: root = [1,2,3,4,5,6] Output: 6
Example 2:
Input: root = [] Output: 0
Example 3:
Input: root = [1] Output: 1
Constraints:
- The number of nodes in the tree is in the range
[0, 5 * 104]
. 0 <= Node.val <= 5 * 104
- The tree is guaranteed to be complete.
Solution
class Solution {
int height(TreeNode *root) {
if(!root) return -1;
int h = 0;
while(root) {
h += 1;
root = root->left;
}
return h-1;
}
public:
int countNodes(TreeNode* root) {
if(!root) return 0;
int h = height(root);
return h < 0 ? 0 :
height(root->right) == h - 1 ? (1 << h) + countNodes(root->right) :
(1 << (h - 1)) + countNodes(root->left);
}
};
// Accepted
// 18/18 cases passed (30 ms)
// Your runtime beats 97.25 % of cpp submissions
// Your memory usage beats 93.85 % of cpp submissions (30.7 MB)