2021-05-22 Daily-Challenge
Today is Saturday, I gonna review the tasks I've done this week, and finish today's leetcode's May LeetCoding Challenge with cpp
.
LeetCode Review
Find and Replace Pattern
too easy to review
Binary Tree Level Order Traversal
too easy to review
Longest String Chain
won't review
Find Duplicate File in System
won't review
Minimum Moves to Equal Array Elements II
class Solution {
public:
int minMoves2(vector<int>& nums) {
int answer = 0;
sort(nums.begin(), nums.end());
int target = nums[nums.size() / 2];
for(auto i : nums) answer += abs(i - target);
return answer;
}
};
May LeetCoding Challenge 22
Description
N-Queens
The n-queens puzzle is the problem of placing n
queens on an n x n
chessboard such that no two queens attack each other.
Given an integer n
, return all distinct solutions to the n-queens puzzle.
Each solution contains a distinct board configuration of the n-queens' placement, where 'Q'
and '.'
both indicate a queen and an empty space, respectively.
Example 1:
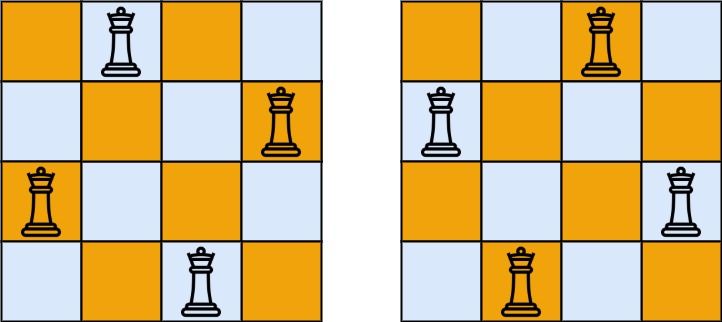
Input: n = 4
Output: [[".Q..","...Q","Q...","..Q."],["..Q.","Q...","...Q",".Q.."]]
Explanation: There exist two distinct solutions to the 4-queens puzzle as shown above
Example 2:
Input: n = 1 Output: [["Q"]]
Constraints:
1 <= n <= 9
Solution
int rows[9];
bool check(int row, int col) {
for(int i = 0; i < row; ++i) {
if(abs(i - row) == abs(rows[i] - col) || col == rows[i]) return false;
}
return true;
}
class Solution {
vector<vector<string>> answer;
void addResult(int n) {
vector<string> result;
for(int i = 0; i < n; ++i) {
string row(n, '.');
row[rows[i]] = 'Q';
result.emplace_back(row);
}
answer.emplace_back(result);
}
void solve(int cur, int n) {
if(cur == n) {
addResult(n);
return;
}
for(int i = 0; i < n; ++i) {
if(check(cur, i)) {
rows[cur] = i;
solve(cur + 1, n);
}
}
}
public:
vector<vector<string>> solveNQueens(int n) {
solve(0, n);
return move(answer);
}
};