2021-05-20 Daily-Challenge
I'm busy playing Dungeons & Fighters, so won't do pick up.
Today I have done leetcode's May LeetCoding Challenge with cpp
.
May LeetCoding Challenge 20
Description
Binary Tree Level Order Traversal
Given the root
of a binary tree, return the level order traversal of its nodes' values. (i.e., from left to right, level by level).
Example 1:
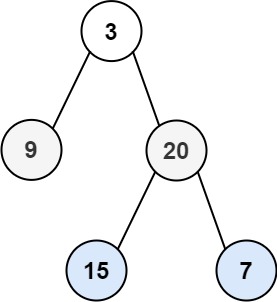
Input: root = [3,9,20,null,null,15,7]
Output: [[3],[9,20],[15,7]]
Example 2:
Input: root = [1]
Output: [[1]]
Example 3:
Input: root = []
Output: []
Constraints:
- The number of nodes in the tree is in the range
[0, 2000]
. -1000 <= Node.val <= 1000
Solution
class Solution {
public:
vector<vector<int>> levelOrder(TreeNode* root) {
if(!root) return {};
queue<TreeNode*> q;
vector<vector<int>> answer;
q.push(root);
while(q.size()) {
int sz = q.size();
vector<int> curLevel;
for(int i = 0; i < sz; ++i) {
auto node = q.front();
q.pop();
curLevel.push_back(node->val);
if(node->left) {
q.push(node->left);
}
if(node->right) {
q.push(node->right);
}
}
answer.push_back(curLevel);
}
return answer;
}
};